There are occasions, when you want to restart failed flow (Power Automate) runs or enabling (turning on) flows, after a solution deployment. You can do either of these tasks manually in the web browser or better still create repeatable scripts, combining PowerShell Cmdlets and the commands from the CLI for Microsoft 365.
- Microsoft 365 CLI . can manage the Microsoft 365 tenant, regardless of which platform (Linux, MacOs Windows and versions of PowerShell: (Windows PowerShell, PowerShell Core) you wish to run the commands in.
- PowerShell support for PowerApps is a series of Cmdlets, available in separate modules for both Administrator and Maker commands. As both modules use the .NET framework, they will only run within Windows PowerShell Session.
QUICK NOTE
Ensure your development and UAT SharePoint Lists have over 5000+ rows during the development and test cycles, to allow testing for List View Threshold, Non delegable and Pagination errors (Power Automate).
Restart Flow Runs
Flows can fail for reasons such as not handling an exception condition correctly or even password or policy changes to a tenant, that can invalidate the access or refresh tokens.
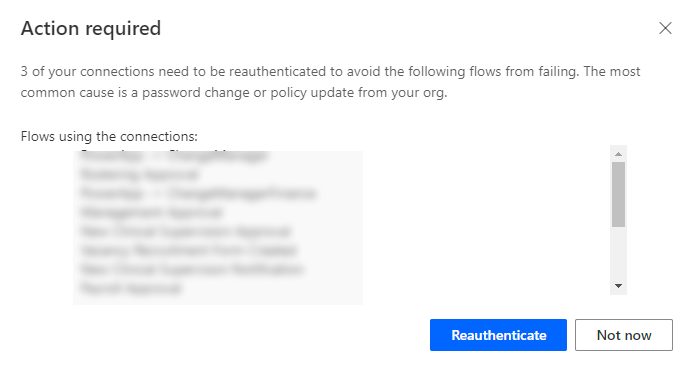
Ideally, you will need to open a private or incognito browser session and login as the owning account for the flow. If this flow is part of a Power Platform solution, then this will be the account used to deploy the solution.
Navigate to https://make.powerapps.com and click on the left hand Flows menu item. Alternatively, you can navigate to https://make.powerautomate.com and click on the left hand My flows menu item.
Click on one or more of the failed flow runs.
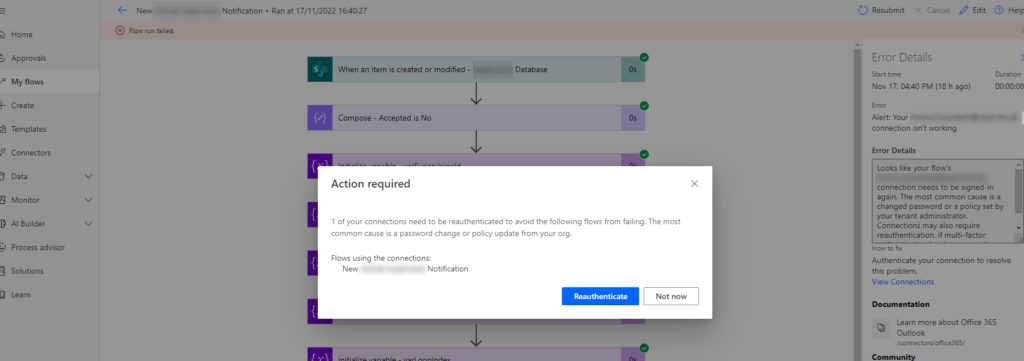
First, you need to view the flow details in the flow environment to locate both Environment Name (Id) and the Flow Name t(Id). These will both be arguments to use in the script.

Mohamed Ashiq Faleel, has submitted really useful community sample: Resubmit all failed flow runs for a flow in an environment | PnP Samples , to find and re-run failed flow runs. Copy and save as a PowerShell (.ps1) file; then call the script, passing in the above Environment Name and Flow Name values.
npm install -g @pnp/cli-microsoft365 m365 login $flowEnvironment = <your environment name> $flowEnvironment = <your flow name> .\rePnPRunFailedFlows.Ps1 $flowEnvironment $flowName
Another approach is to query the Environment Display Name .e.g “mytenant (default)” to find the Environment Name (Object Id). Likewise, query the Flow Display Name .e.g “my handy flow” to find the Flow Name (Object Id). The CLI for Microsoft 365 allows query expressions to be written using the JMESPath syntax to handle these types of operations and also filter returned datasets, returned when you run a command. In the JMESPath query, you can include hard-coded values, e.g. "[?status =='Failed']"
. Alternatively, build up the query expression dynamically with a variable, embedding single quotes around its literal value.
Script to build query expressions dynamically
$envDisplayName = "mydevtenant (default)" if (![String]::IsNullOrEmpty($envDisplayName)) { #build query for m365 parameter ensuring we embed the single quotes around the value $query = "[?displayName == '"+ $envDisplayName +"']" $flowEnvironmentJson = (m365 flow environment list --query $query) #convert the single item json array $flowEnvironment = ($flowEnvironmentJson | Convertfrom-Json).name } $flowDisplayName = "SPO->Gen Purpose List new or modified item" if (![String]::IsNullOrEmpty($flowDisplayName)) { #build query for m365 parameter ensuring we embed the single quotes around the value $query = "[?displayName == '"+ $flowDisplayName +"']" $flowJson = (m365 flow list --environment $flowEnvironment --query $query) #convert the single item json array $flowName = ($flowJson | Convertfrom-Json).name } Write-host "Environment Name " $flowEnvironment Write-host "Flow Name " $flowName
If you want to list failed flow runs after the date and time shown in then, then amend the above script to include the status and startTime property values in your JMESPath query. Note, the startTime or endTime properties must use the ISO 8601 Date and Time format in the query regardless of the date display format shown.
$flowRuns = m365 flow run list --environment $flowEnvironment --flow $flowName ` --query "[?status =='Failed' && properties.startTime > '2022-11-03T16:27:49.50301Z']"
The query will exclude the item below from the returned dataset as it occurs a few seconds before the specified startTime value.
Run details: name : 08585341140283153191273269301CU25 id : /providers/Microsoft.ProcessSimple/environments/b59d1516-4a42-48ff-b892-daa2b8942fe8/flows/923e84f5-05b5-46c0-af7d-13d94a a7f3c9/runs/08585341140283153191273269301CU25 type : Microsoft.ProcessSimple/environments/flows/runs properties : @{startTime=03/11/2022 16:27:37; endTime=03/11/2022 16:27:48; status=Failed; code=Terminated; error=; correlation=; trigger=} startTime : 03/11/2022 16:27:37 status : Failed
Re-enable Flows
Depending on your deployment approach for importing a managed solution: Manually, through the browser or a CD/CI (DevOps) deployment pipeline, into a target environment, you may need an additional step to enable one or more flows in your solution. If you create a script to enable the flows as part of the deployment process, then you an additional error checking step to valid any required connections or permissions are in place, before the flow will enable.
Script to install the Microsoft PowerShell Modules in a Windows PowerShell Session
If you want to just get the flows created by a specific user, e.g. the service account used to deploy a solution, then there are additional steps needed to locate the user’s Object Id held in Azure AD.
# Perform a quick check to ensure that you are running the script in a Windows PowerShell session. If ($PSVersionTable.PSVersion.Major -gt 5.0){ throw ( "You need to run this script under Windows PowerShell 5.1x as the modules require the .NET framework") } else { Install-Module -Name Microsoft.PowerApps.Administration.PowerShell -AllowClobber -Force -Scope CurrentUser Install-Module -Name Microsoft.PowerApps.PowerShell -AllowClobber -Force -Scope CurrentUser } # Login as the System Admin for the target environment, so we can run the admin Cmdlets later in the script Add-PowerAppsAccount -Username $User
Locate only the flows created by a specified user
Installing the additional Microsoft Graph PowerShell Module
s
gives you Cmdlets to access the Microsoft Graph APIs from services such as Azure AD. Exchange, SharePoint et al. These modules are cross platform and can run from either WIndows PowerShell or PowerShell (Core) session. As shown below, connecting to Microsoft Graph with a minimal permission scope allows you to locate a user’s Object ID, though a search against the User principal name (email address). We then pass the Object Id as an argument to the Get-AdminFlow
command to only find flows in the target environment created by the user. Omit the UserId parameter in the Get_AdminFlow
command, if you want to locate all flows in the environment.
# List the flows created by a spefic user then Install-Module Microsoft.Graph -Scope CurrentUser Connect-MgGraph -Scopes "User.Read.All","Group.ReadWrite.All" $flowCreatedUser = "MeganB@coder365.onmicrosoft.com" $flowCreatedUserId = (Get-Mguser -UserId $flowCreatedUser).Id # Add the argument to just locate the flows created by the above user $deployedFlows = Get-AdminFlow -EnvironmentName $envName -CreatedBy $flowCreatedUserId #.... # Also display the email of the user If($response -eq "Y") { Enable-AdminFlow -EnvironmentName $envName -FlowName $flow.FlowName Write-Host -fore DarkGreen "Flow: " $flow.DisplayName "Created By " $flowCreatedUser "enabled sucessfully!" }
If the flow enables successfully, then happy days, you will get a similar message to the one shown blow. Otherwise, you will see an exception raised.

Hopefully, this post has shown how easy it is to restart failed flow runs or reenable flows with the CLI for Microsoft and the Power Platform PowerShell Modules. Please let me know your thoughts in the comments.